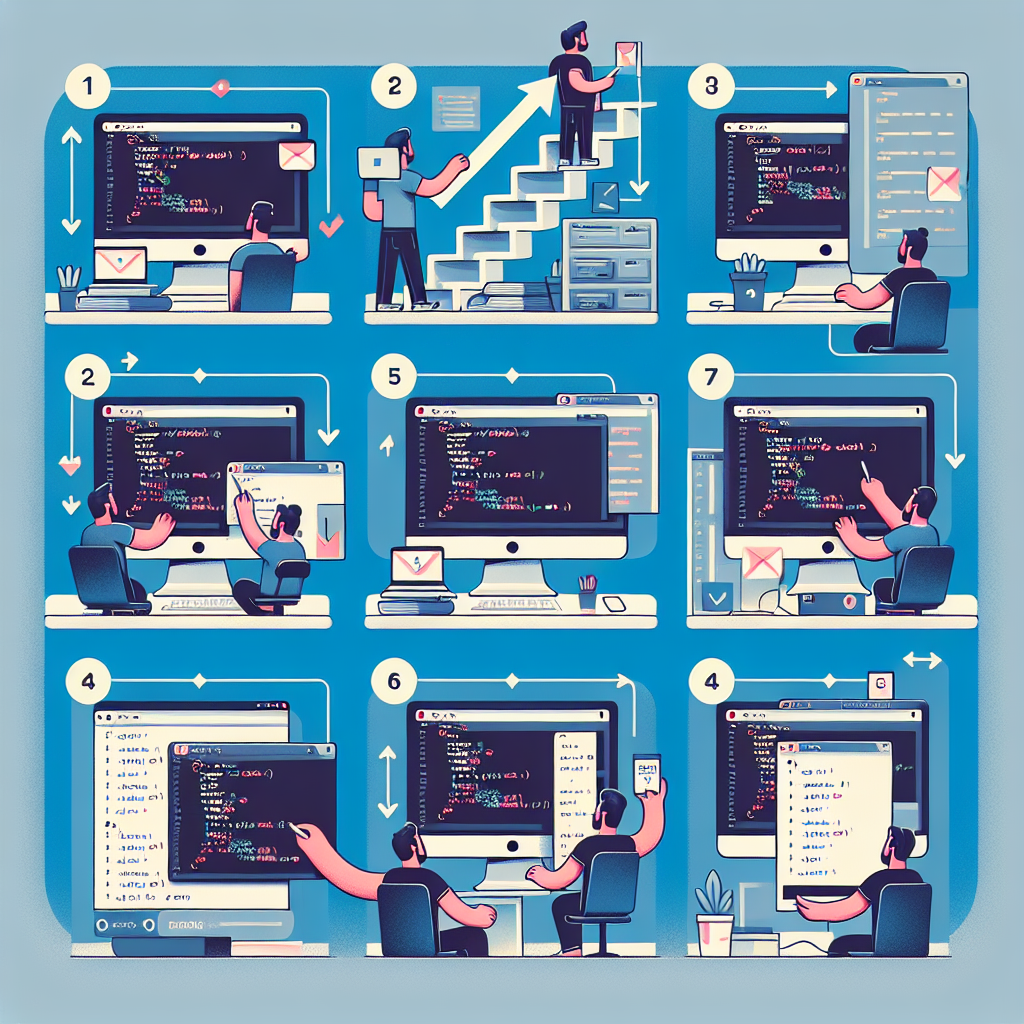
In this tutorial, I'm going to show you how to write a simple chat application using MeteorJS.
Here is a live demo as our goal: http://hrr2demo.meteor.com/
And the source code on GitHub: https://github.com/victorleungtw/hrr2demo
Feel free to try the demo and fork the repo before we get started.
What is Meteor?
Meteor is an awesome web framework for building real-time applications using JavaScript. It is based on NodeJS and MongoDB.
Step 1: Install Meteor
For macOS and Linux users, run the following command in your terminal:
> curl [https://install.meteor.com/](https://install.meteor.com/) | sh
Step 2: Create a New App
> meteor create awesomeChatApp
Then change the directory to your app:
> cd awesomeChatApp
Try to run it:
> meteor
And open your browser at the address: http://localhost:3000
Step 3: Create Folders and File Structure
Remove the default files with the command:
> rm awesomeChatApp.*
Create three folders:
> mkdir client server both
By convention, we'll put anything that runs on the client side (i.e., the user's browser) in the 'client' folder, anything that runs on the server side in the 'server' folder, and anything accessed by both client and server in the 'both' folder.
Step 4a: Create a Collection to Store Messages
Inside the 'both' folder, we'll place our model here.
> touch collection.js
To create a new 'messages' collection:
Messages = new Meteor.Collection("messages")
Step 4b: Create the Index Page
Inside the 'client' folder, we'll place our homepage view.
> touch index.html
With the following HTML:
<head>
<title>chatterbox</title>
</head>
<body>
{{> loginButtons align="right"}} # chatterbox {{> input}} {{> messages}}
</body>
Step 4c: Create HTML Template with Helpers
To better organize our files, we can create a new folder:
> mkdir messages > cd messages > touch messages.html
Now, we create a template with the name 'messages'.
<template name="messages">
{{#each messages}} {{name}}: {{message}} {{/each}}
</template>
And we'll create helpers to loop through each message in the 'Messages' collection.
Template.messages.helpers({
messages: function () {
return Messages.find({}, { sort: { time: -1 } })
},
})
Step 4d: Create HTML Template with Event Handlers
Similarly, we'll create a template for the input box and submit button.
> mkdir input > cd input > touch input.html
<template name="input">
<form id="send">
<input id="message" type="text" />
<input type="submit" value="Submit" />
</form>
</template>
Also, we'll create a JavaScript file to handle the click event of the submit button.
Template.input.events({
"submit form": function (event) {
event.preventDefault()
var name = Meteor.user() ? Meteor.user().profile.name : "Anonymous"
var message = document.getElementById("message")
if (message.value !== "") {
Messages.insert({
name: name,
message: message.value,
time: Date.now(),
})
message.value = ""
}
},
})
Step 4e: Add Accounts Package for Login Using GitHub Account
Meteor is very easy to use. Because it has a rich package ecosystem, we're going to add accounts-ui
and accounts-github
for a simple user login system.
meteor add accounts-ui
meteor add accounts-github
Step 4f: Add Stylesheets (Optional)
Copy and paste the following stylesheets inside your 'client' folder.
> mkdir style > cd style > touch style.css
Step 5: Deploy Your Website to Meteor's Free Server
meteor deploy awesomeChatApp.meteor.com
Done! You may need to deploy to a different address if the given address is already in use. Open your browser with your address: http://awesomeChatApp.meteor.com
You will also need to configure the login system