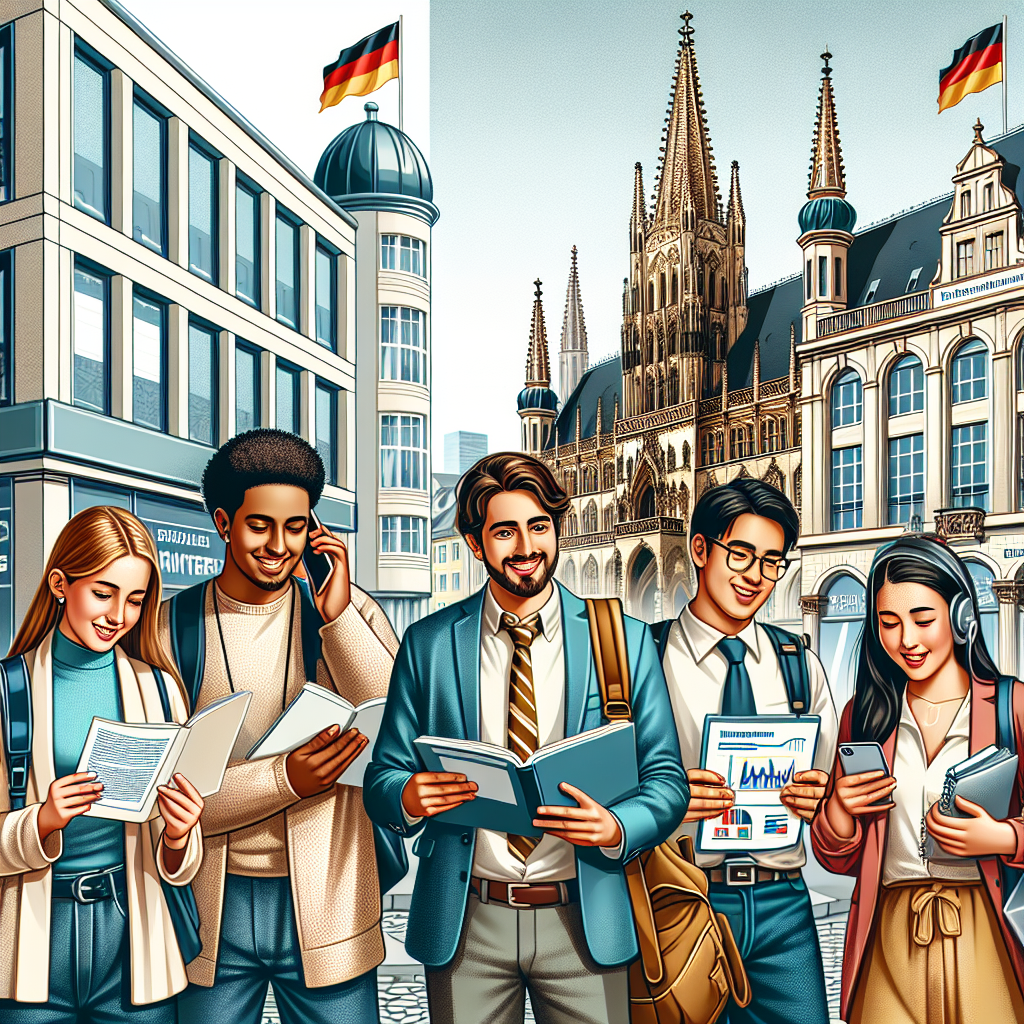
Traveling involves a great deal of uncertainty and chance. Despite the delay of my flight in Helsinki, Finland, and the stress from a jet lag-induced presentation, the journey proved worthwhile as it enriched my professional management skills beyond the classroom.
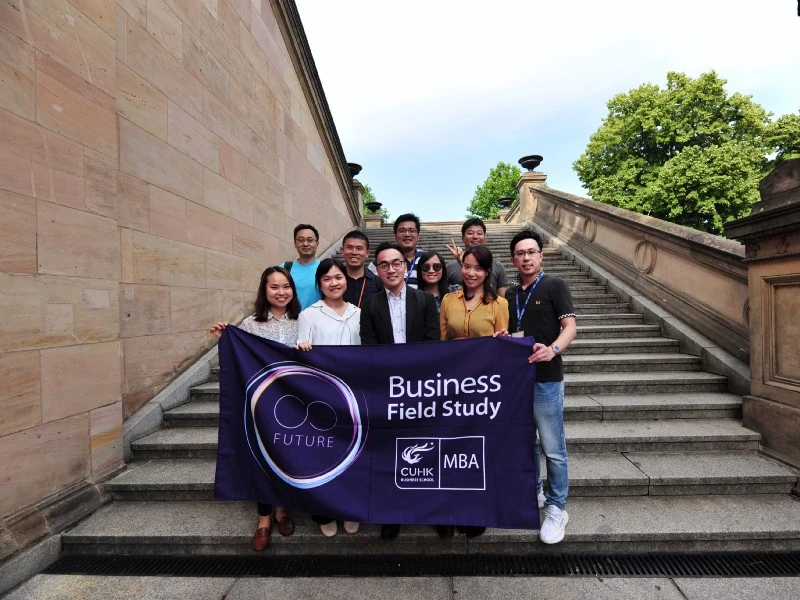
The tour was filled with thought-provoking and educational talks on topics such as Germany's macroeconomics, the European Union, hidden champions, Industry 4.0, and artificial intelligence. Our visits to Wattx, a deep tech business, and the Daimler AG factory, where BMW automobiles are manufactured, were enlightening. The most significant personal takeaway from the trip is that Germany is antifragile. Although Germany was fragile during World War II, its current wealth demonstrates its antifragility.
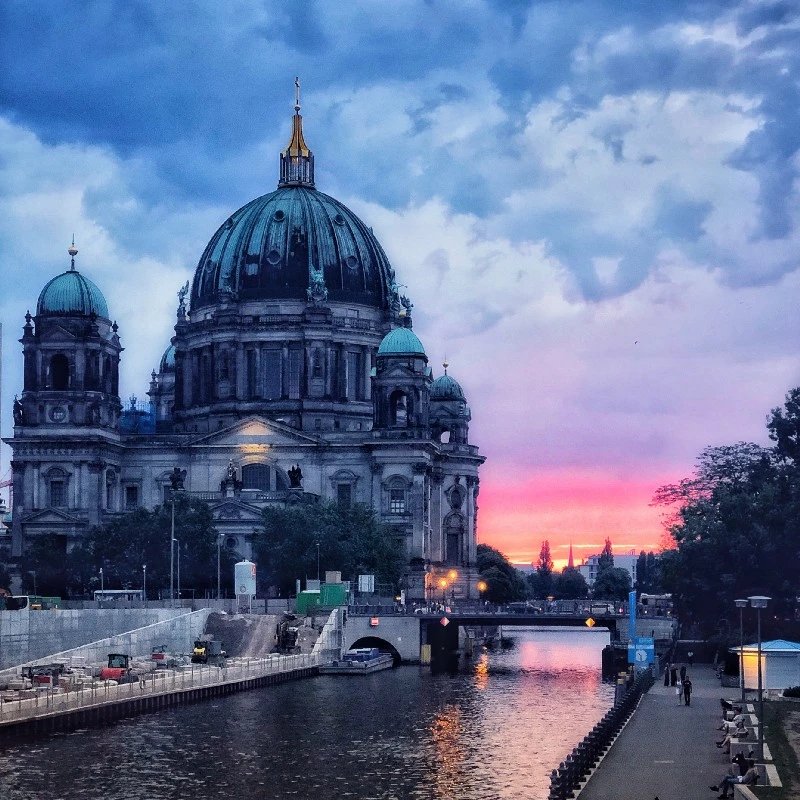
The trip revealed that Germany has the highest number of hidden champions—companies that are either in the global top three or the number one in Europe, generate annual revenues of more than 5 million euros, and maintain a low degree of public recognition. With numerous small-to-medium enterprises (SMEs) specializing in deep technology, the German economy thrives on exports and innovation. These family-owned enterprises are clustered and decentralized across Germany rather than being concentrated in Berlin, among the 1,307 hidden champions.

From my perspective, Germany is antifragile due to its vibrant start-up scene and decentralization. Large corporations may have strong hierarchical structures and numerous regulations, but they are vulnerable due to higher turnover rates and lesser adaptability and flexibility. SMEs, on the other hand, boast lean structures that foster a high-performance culture, increased employee engagement, and a more people-oriented approach. Such antifragility also manifests in more frequent employee transfers between various functions within the business. Employees at SMEs generally have more direct customer contact, enabling them to better understand their clients and respond to market shifts more quickly.

To understand how these hidden champions remain competitive, one must recognize their specialization in specific market categories. They excel at creating premium quality products in niche markets and possess a global reach. These companies invest heavily in Research and Development (R&D), and their culture of continuous innovation is fueled by both customers and top executives. All these elements contribute to their antifragility, allowing them to thrive and grow despite global market instability and unpredictability.

I noticed a distinct difference in mentality between Germany and Hong Kong. While most students in Hong Kong gravitate towards careers in the finance and banking industries, Germany offers a broader range of sectors like steel, iron, machinery, chemicals, locomotives, automobiles, and electronics. Rather than viewing university education as the only career path, Germany provides more vocational training for technical skills. The diverse job opportunities and emphasis on manufacturing and technological innovation make these countries less susceptible to risks.
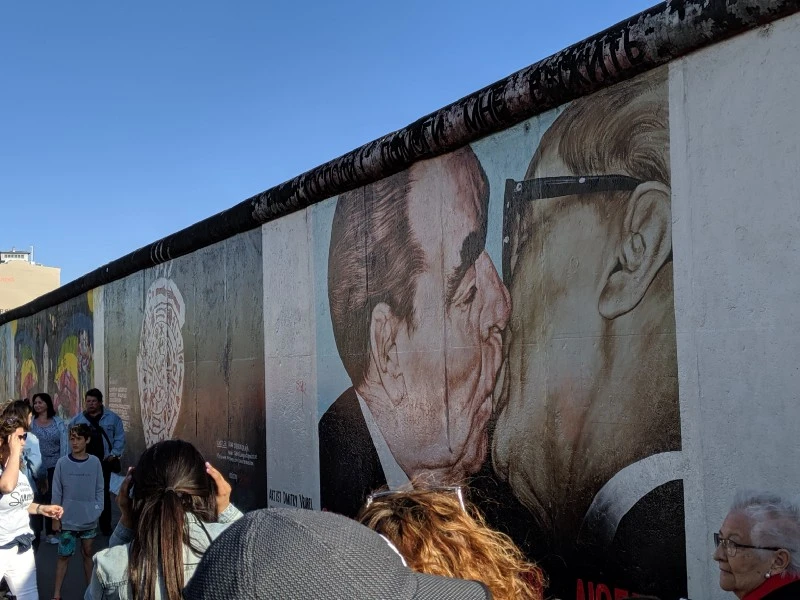
Germany's economy is stable, characterized by low inflation, steady growth, a trade surplus, and a robust labor force. Effective state management helps in avoiding the recurrence of economic crises like the Great Depression and hyperinflation that contributed to the rise of Nazism and political instability in World War II. Understanding history is crucial to comprehending our origins, current challenges, and improving risk management in chaotic situations.
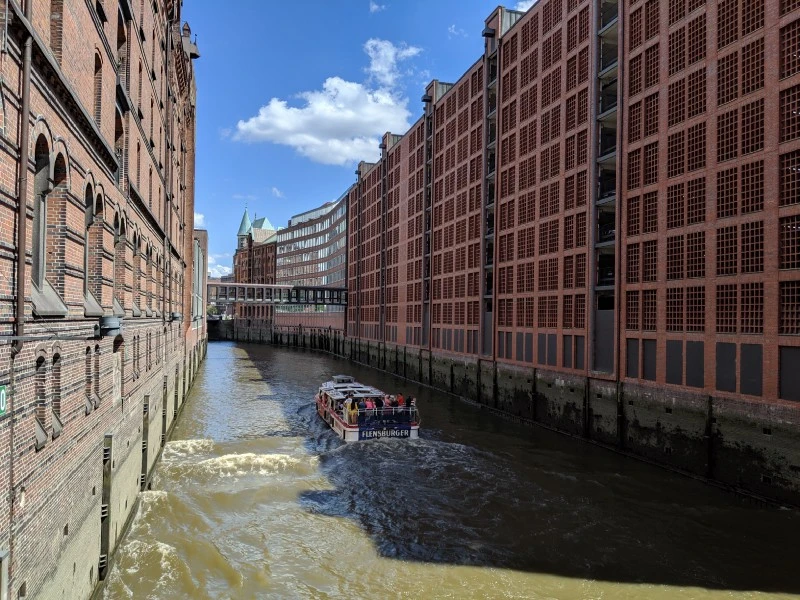
One of the main reasons I pursued an MBA was to refine my management skills in the global market. I was intrigued by the significant disparities in wealth between countries. For instance, after my study trip, I visited Switzerland and found myself eating the most expensive McDonald's Big Mac meal I've ever had, costing 12 Swiss Francs (approximately $93 in Hong Kong dollars). In contrast, a similar meal costs 7 Euros in Germany or roughly $62 in Hong Kong. The world can seem unjust if you're born in an economically weaker Eurozone country, such as rural Romania, where human trafficking is a severe issue. This journey allowed me to witness the elements of Germany's success and its antifragility throughout my visits to Berlin, Hamburg, Munich, and Frankfurt.
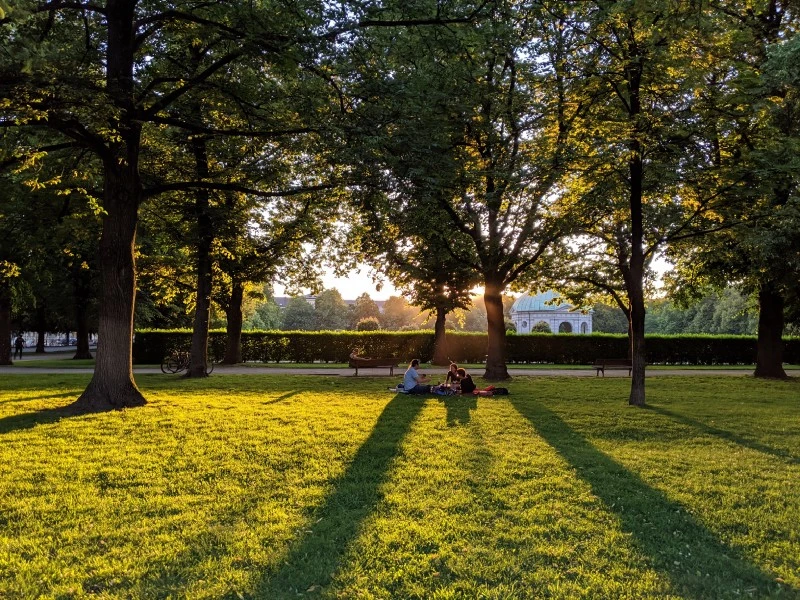
The lessons learned will serve me well as I aim to create an antifragile workplace at the management level. In today's rapidly changing digital environment, it's essential to build a company capable of thriving in unpredictability and uncertainty, beyond mere resilience or robustness. As I've observed in Germany, smaller, flat teams are antifragile, while large hierarchical structures are unstable. Antifragile organizations embrace a culture of experimentation and shun the "too-big-to-fail" mentality. In such settings, managers are wary of centralized power, and leaders trust their teams to handle complex challenges through decentralized decision-making.

Living in the age of artificial intelligence and automation is exhilarating. Our industry is undergoing a profound transformation, with an expanding horizon of untapped opportunities, increasingly connected devices, and more available real-world data. After completing my MBA, I continue to strive for greater antifragility in this fast-changing world, fortified by the unique perspectives gained from my international experiences in Germany.
